一、注解使用模式
-
@EnableCaching:在主入口类上添加该注解,用于开启缓存;
-
@CacheConfig:标注在类上,表示当前类使用的缓存组件中的key为该注解指定的cacheNames/value,当该注解指定了cacheNames/value之后,@Cacheable上就无需再使用cacheNames/value了;
-
@Cacheable:将方法的结果进行缓存;
-
cacheNames/value:缓存组件的名字;
-
key:缓存数据使用的key,默认是使用方法参数的值,可以使用SpEL表达式,比如#id == #a0 == #p0 == #root.args[0]都表示使用第一个参数的值作为缓存的key;
-
keyGenerator:key的生成器,可以自己指定key的生成器的组件id;
-
cacheManager:指定缓存管理器;
-
cacheResolver:指定获取解析器;
-
condition:指定符合条件的情况下才缓存,支持SpEL表达式;
- condition=“#id>1”:表示id的值大于1时才缓存。
-
unless:否定缓存,当unless指定的条件为true,方法的返回值就不会被缓存,该属性可以获取到结果进行判断,unless与condition刚好相反;
- unless=“#a0==2”:表示第一个参数的值为2时,查询结果不缓存。
-
sync:是否使用异步模式,使用sync后unless就不支持了;
其中keyGenerator与key二者只能选一,cacheResolver与cacheManager也二者选一。
-
-
@CachePut:用于更新缓存,它先调用方法,然后将目标方法的结果进行缓存。
能够更新缓存的前提是,@CachePut更新使用的key与@Cacheable使用的key要保持一致。
@CachePut注解属性同@Cacheable的属性类似,少了sync属性。
-
@CacheEvict:缓存清除
同样如果要清除缓存,则使用的key值要与@Cacheable使用的key一致,否则达不到清除缓存的功能。
@CacheEvict注解属性比@Cacheable注解少了sync和unless,但是多了两个属性:allEntries和beforeInvocation。
allEntries:表示是否删除所有缓存。
beforeInvocation:表示删除缓存的操作是否在目标方法执行之前。
@Cacheable、@CachePut、@CacheEvict
- @Cacheable就是用来替换我们原来的判空,查询缓存并返回的操作;
- @CachePut则是每次都会执行方法,其实只是有一个存入缓存的操作(只是“put”);
- @CacheEvict 是用来清空缓存的。
注解使用
@RedisString(key = "user:${userId}")
public class User {
private int userId;
private String userName;
private String password;
// getters and setters
}
Redis String类型注解使用@RedisString注解实现,它可以将Java对象直接映射到Redis中的String类型。在Java代码中使用时,只需要通过注解标注一下对应的Key,就能将数据存储到Redis中。
@RedisList(key = "user:list")
public class UserList {
private List userList;
// getters and setters
}
这里通过@RedisList注解将UserList对象映射到Redis中的"user:list",将User对象存储到Redis的List中。当程序需要获取所有用户信息时,只需要从Redis中获取"user:list"对应的List对象,就能获取到所有User对象。
@RedisSet(key = "user:set")
public class UserSet {
private Set userSet;
// getters and setters
}
这里通过@RedisSet注解将UserSet对象映射到Redis中的"user:set",将User对象存储到Redis的Set中。当程序需要获取所有用户信息时,只需要从Redis中获取"user:set"对应的Set对象,就能获取到所有User对象。
@RedisSortSet(key = "user:sort", scoreName = "score")
public class UserSortSet {
private int score;
private User user;
// getters and setters
}
这里通过@RedisSortSet注解将UserSortSet对象映射到Redis中的"user:sort",并将User对象存储到Redis的SortedSet中。scoreName用于指定分值所对应的属性。
当程序需要获取排名前n的用户信息时,只需要从Redis中获取"user:sort"对应的SortedSet对象,使用zrangeWithScores方法获取排名前n的UserSortSet对象。其实现方法如下:
Set tuples = jedis.zrangeWithScores("user:sort", 0, n - 1);
for (Tuple tuple : tuples) {
UserSortSet userSortSet = new UserSortSet();
userSortSet.setUser(jedis.hget("user:${userId}", "userName"));
userSortSet.setScore(tuple.getScore());
userSortSets.add(userSortSet);
}
@RedisHash(key = "user", field = "userId")
public class UserHash {
private int userId;
private String userName;
private String password;
// getters and setters
}
这里通过@RedisHash注解将UserHash对象映射到Redis中的"user",将属性userId作为field,将User对象存储到Redis的Hash中。
当程序需要获取某一个用户信息时,只需要从Redis中获取"user"对应的Hash对象,并使用hget方法获取对应field的值即可。
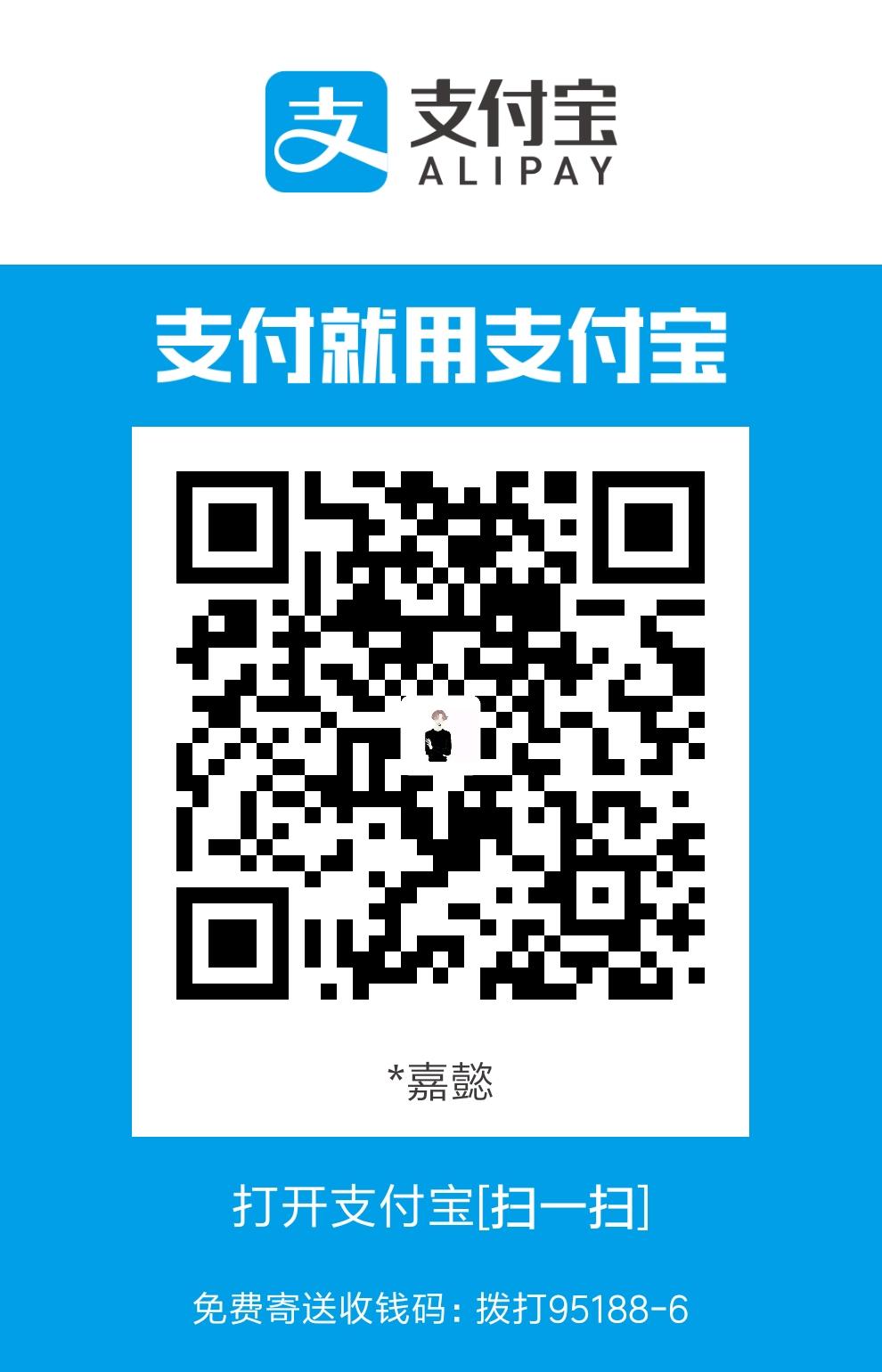
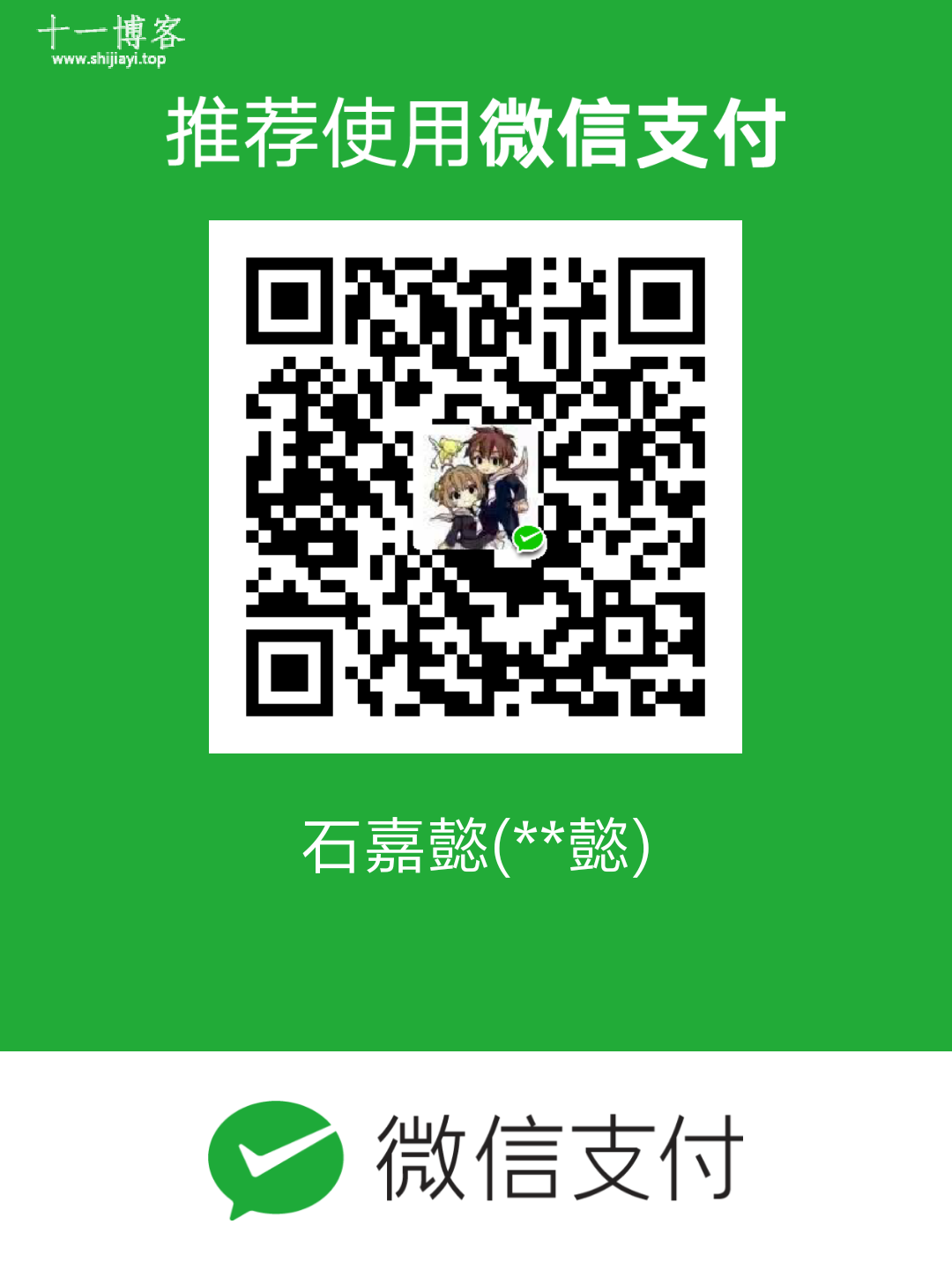