/**
* Shiro登录接口
*/
@ApiOperation(value = "Shiro登录接口", notes = "Shiro登录接口")
@PostMapping("/loginShiro")
public Result<Map<String,Object>> loginShiro(String userName, String passWord) {
//shiro验证
Subject subject= SecurityUtils.getSubject();
//根据用户名密码生成一个令牌
UsernamePasswordToken token=new UsernamePasswordToken(userName,passWord);
try {
subject.login(token); //执行登录操作
} catch (UnknownAccountException e) {
log.info("登录用户不存在");
return Result.error(416,"用户不存在");
} catch (IncorrectCredentialsException e) {
log.info("登录密码错误");
return Result.error(412,"密码错误,请重新登录");
}catch (AuthenticationException e) {
log.warn("用户登录异常:" + e.getMessage());
return Result.error(416,"账户异常");
}
return Result.ok();
}
package com.xxgc.springboot.realm;
import com.xxgc.springboot.entity.Users;
import com.xxgc.springboot.service.IUsersService;
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.authc.*;
import org.apache.shiro.authz.AuthorizationInfo;
import org.apache.shiro.authz.SimpleAuthorizationInfo;
import org.apache.shiro.crypto.hash.Md5Hash;
import org.apache.shiro.realm.AuthorizingRealm;
import org.apache.shiro.session.Session;
import org.apache.shiro.subject.PrincipalCollection;
import org.apache.shiro.util.ByteSource;
import org.springframework.beans.factory.annotation.Autowired;
import java.util.HashSet;
/**
* @program: springboot
* @description: 鉴权
* @author: Mr.abel(ShiJiaYi)
* @create: 2022-09-28 10:24
**/
public class UserRealm extends AuthorizingRealm {
@Autowired
private IUsersService ius;
//去获取到授权的信息
//获取当前用户角色用户、权限信息
@Override
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principalCollection) {
//通过用户id去拿角色,通过角色拿到权限
Integer id = (Integer) SecurityUtils.getSubject().getSession().getAttribute("id");
HashSet<String> perms = new HashSet<>();
perms.add("users:/users/loginShiro");
SimpleAuthorizationInfo info = new SimpleAuthorizationInfo();
info.setStringPermissions(perms);
return info;
}
//从数据库获取当前用户的账号、密码信息(认证)
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException {
// 第一种方式
// 获取用户输入的账号和密码(一般只需要获取账号就可以)
String username = (String) token.getPrincipal();
//String password = new String((char[]) token.getCredentials());
// 通过username从数据库中查找 User对象,如果找到则进行验证
Users users = new Users();
users.setUname("123");
users.setUpass("9cf40d96509912befa03868676b2aa9c");
// 判断账号是否存在
if (users == null){
throw new AuthenticationException();
}
// 判断账号是否被冻结
/*if (users.getState() ==null || user.getState().equals("PROHIBIT")){
throw new LockedAccountException();
}*/
// 进行验证
SimpleAuthenticationInfo authenticationInfo = new SimpleAuthenticationInfo(
// 用户名
users.getUname(),
// 密码
users.getUpass(),
// 盐值
ByteSource.Util.bytes("admin8d78869f470951332959580424d4bf4f"),
//ByteSource.Util.bytes(user.getSalt()),
getName()
);
SecurityUtils.getSubject().getSession().setAttribute("id",1);
return authenticationInfo;
}
public static void main(String[] args) {
Md5Hash md5Hash = new Md5Hash("321","admin8d78869f470951332959580424d4bf4f",2);
System.out.println(md5Hash);
}
}
package com.xxgc.springboot.config;
import com.xxgc.springboot.realm.UserRealm;
import org.apache.shiro.authc.credential.HashedCredentialsMatcher;
import org.apache.shiro.mgt.SecurityManager;
import org.apache.shiro.spring.security.interceptor.AuthorizationAttributeSourceAdvisor;
import org.apache.shiro.spring.web.ShiroFilterFactoryBean;
import org.apache.shiro.web.mgt.DefaultWebSecurityManager;
import org.springframework.aop.framework.autoproxy.DefaultAdvisorAutoProxyCreator;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* @program: springboot
* @description: shiro的配置
* @author: Mr.abel(ShiJiaYi)
* realm:域对象,用来访问数据库得到用户账号、密码、角色、权限信息等。需要程序员自己实现
* securityManager:安全管理器,整合shiro的核心,赋值校验账号密码、权限等操作
* 过滤器:用来指定哪些请求,资源需要认证或者授权(需要哪些数据)
**/
@Configuration
public class ShiroConfiguration {
//此处用于实现授权功能,配置需要拦截的接口
@Bean
public ShiroFilterFactoryBean getShiroFilterFactoryBean(@Qualifier("getDefaultWebSecurityManager") DefaultWebSecurityManager defaultWebSecurityManager) {
ShiroFilterFactoryBean shiroFilterFactoryBean = new ShiroFilterFactoryBean();
shiroFilterFactoryBean.setSecurityManager(defaultWebSecurityManager);
//拦截页面
Map<String, String> map = new LinkedHashMap<>();
//登录/登出,所有人的权限
map.put("/users/loginShiro","anon");
//其他需要权限验证
map.put("/**","authc");
shiroFilterFactoryBean.setFilterChainDefinitionMap(map);
//未登录页面跳转
shiroFilterFactoryBean.setLoginUrl("/user/show");
//未有权限页面跳转
shiroFilterFactoryBean.setUnauthorizedUrl("/user/unauthorized");
return shiroFilterFactoryBean;
}
//注入对应的userRealm类
@Bean
public DefaultWebSecurityManager getDefaultWebSecurityManager(@Qualifier("userRealm") UserRealm userRealm) {
DefaultWebSecurityManager SecurityManager = new DefaultWebSecurityManager();
SecurityManager.setRealm(userRealm);
return SecurityManager;
}
@Bean
public UserRealm userRealm() {
UserRealm userRealm = new UserRealm();
//注册MD5加密
userRealm.setCredentialsMatcher(hashedCredentialsMatcher());
return userRealm;
}
/**
* 设置shiro加密方式
*
* @return HashedCredentialsMatcher
*/
@Bean
public HashedCredentialsMatcher hashedCredentialsMatcher(){
HashedCredentialsMatcher hashedCredentialsMatcher = new HashedCredentialsMatcher();
// 使用md5 算法进行加密
hashedCredentialsMatcher.setHashAlgorithmName("md5");
// 设置散列次数: 意为加密几次
hashedCredentialsMatcher.setHashIterations(2);
return hashedCredentialsMatcher;
}
/**
* 开启Shiro的注解(如@RequiresRoles,@RequiresPermissions)
* 配置以下两个bean(DefaultAdvisorAutoProxyCreator和AuthorizationAttributeSourceAdvisor)即可实现此功能
* @return
*/
@Bean
public DefaultAdvisorAutoProxyCreator advisorAutoProxyCreator(){
DefaultAdvisorAutoProxyCreator advisorAutoProxyCreator = new DefaultAdvisorAutoProxyCreator();
advisorAutoProxyCreator.setProxyTargetClass(true);
return advisorAutoProxyCreator;
}
@Bean
public AuthorizationAttributeSourceAdvisor authorizationAttributeSourceAdvisor(SecurityManager securityManager){
AuthorizationAttributeSourceAdvisor authorizationAttributeSourceAdvisor = new AuthorizationAttributeSourceAdvisor();
authorizationAttributeSourceAdvisor.setSecurityManager(securityManager);
return authorizationAttributeSourceAdvisor;
}
}
最后修改于 2022-09-29 10:04:33
如果觉得我的文章对你有用,请随意赞赏
扫一扫支付
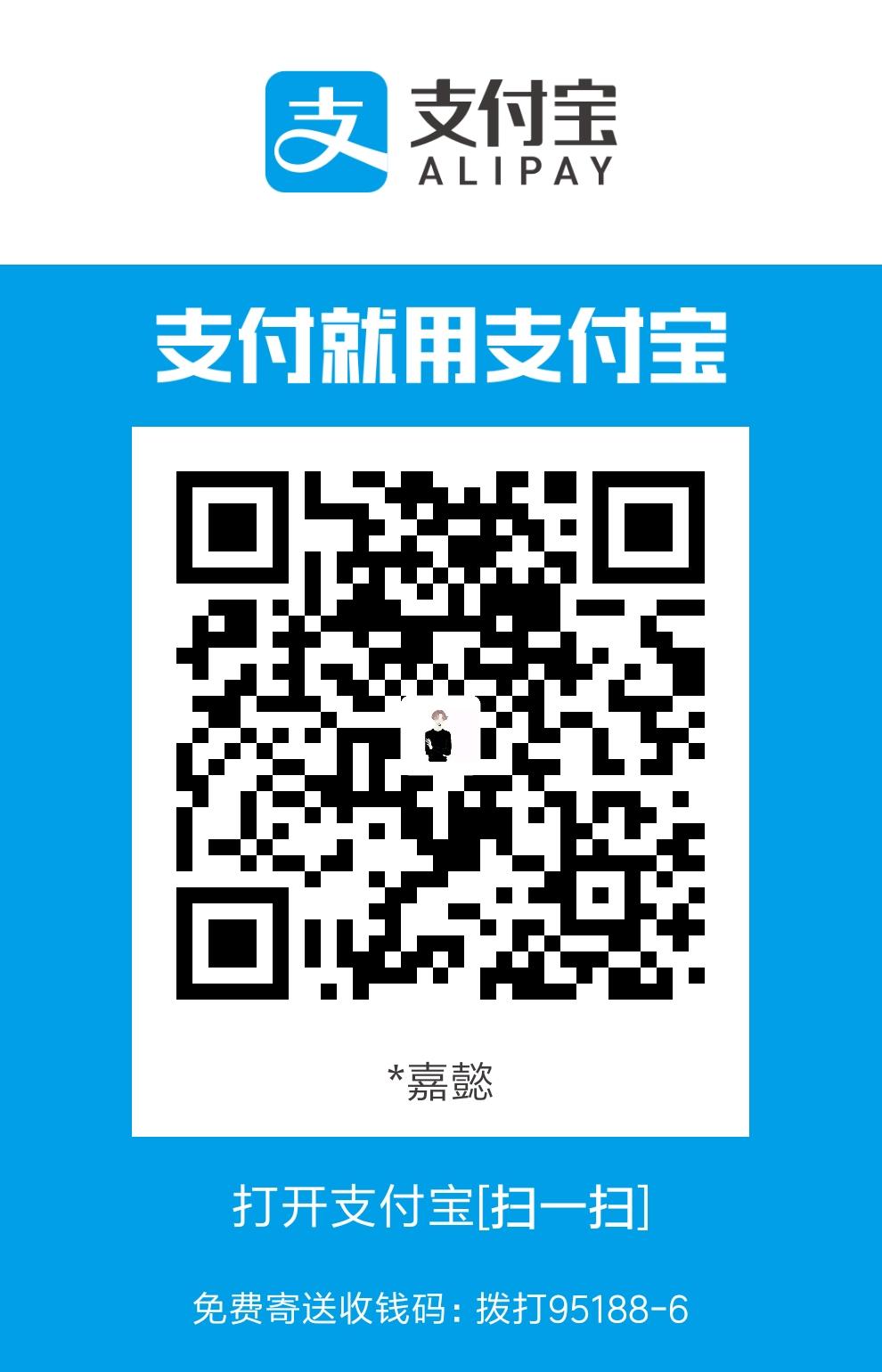
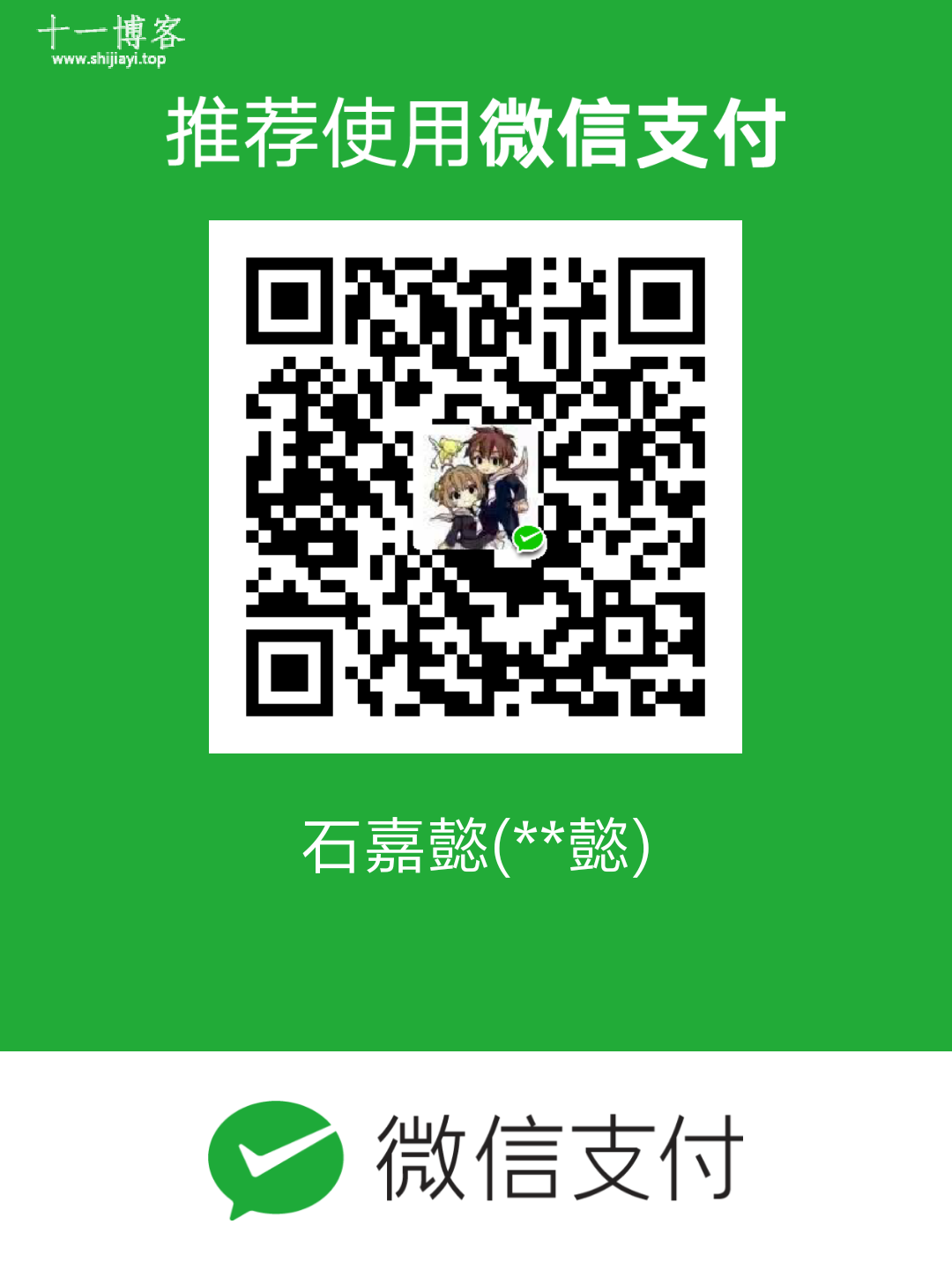