业务逻辑层接口
package com.sjy.automation.service;
import com.sjy.automation.bean.Info;
import com.sjy.automation.bean.RunInfo;
import com.sjy.automation.bean.Step;
public interface IStepService {
//添加步骤1-3
Info addStep(Step si);
//获取所有步骤
Info getStep();
//删除某个步骤
Info delStepOne(Integer id);
//调用Python运行脚本
Info runStep(RunInfo ri);
//停止脚本
Info runStop();
//删除所有运行脚本
Info delStep();
}
脚本业务逻辑
package com.sjy.automation.service.impl;
import com.google.gson.Gson;
import com.google.gson.JsonSyntaxException;
import com.google.gson.reflect.TypeToken;
import com.sjy.automation.bean.Info;
import com.sjy.automation.bean.MegInfo;
import com.sjy.automation.bean.RunInfo;
import com.sjy.automation.bean.Step;
import com.sjy.automation.service.IStepService;
import com.sjy.automation.step.StepRun;
import com.sjy.automation.util.FileUtils;
import com.sjy.automation.util.JsonFileUtils;
import com.sjy.automation.ws.MyWebSocketHandler;
import org.springframework.stereotype.Service;
import java.io.File;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.List;
/**
* @Author 石嘉懿
* 脚本业务逻辑
*/
@Service
public class StepServiceImpl implements IStepService {
private final static String fileUrl = FileUtils.getTomcatPath().substring(1)+"/upload/";
private final static String fileName = "step.txt";
private Gson gson = new Gson();
//脚本线程
private static StepRun sr;
@Override
public Info addStep(Step si) {
Info info = new Info();
try {
//如果目录不存在就创建
JsonFileUtils.mkdirs(fileUrl);
//判断文件是否存在 不存在就创建
if(!JsonFileUtils.isExists(fileUrl+fileName)){
File writename = new File(fileUrl+fileName);
writename.createNewFile(); // 创建新文件
}
//读取文件内容
String content = JsonFileUtils.readText(fileUrl+fileName);
//内容转换为对象
Type type = new TypeToken<List<Step>>(){}.getType();
//步骤集合
List<Step> list = gson.fromJson(content, type);
if(list == null){
list = new ArrayList<Step>();
si.setId(list.size()+1);
}else{
si.setId(list.get(list.size()-1).getId()+1);
}
//添加内容
list.add(si);
String s = gson.toJson(list);
//内容写入到文件
JsonFileUtils.writeText(fileUrl+fileName,s,false);
info.setData(list);
info.setMsg("ok");
info.setCode(200);
} catch (JsonSyntaxException | IOException e) {
info.setCode(-202);
info.setMsg("JSON文件操作异常,请重试");
JsonFileUtils.writeText(fileUrl+fileName,"",false);
}
return info;
}
@Override
public Info getStep() {
Info info = new Info();
try {
//如果目录不存在就创建
JsonFileUtils.mkdirs(fileUrl);
//判断文件是否存在 不存在就创建
if(!JsonFileUtils.isExists(fileUrl+fileName)){
File writename = new File(fileUrl+fileName);
writename.createNewFile(); // 创建新文件
}
//读取文件内容
String content = JsonFileUtils.readText(fileUrl+fileName);
//内容转换为对象
Type type = new TypeToken<List<Step>>(){}.getType();
//步骤集合
List<Step> list = gson.fromJson(content, type);
if(list == null){
list = new ArrayList<Step>();
}
info.setData(list);
info.setMsg("删除完成");
info.setCode(200);
} catch (JsonSyntaxException | IOException e) {
info.setCode(-202);
info.setMsg("JSON文件操作异常");
}
return info;
}
@Override
public Info delStepOne(Integer id) {
Info info = new Info();
//读取文件内容
String content = JsonFileUtils.readText(fileUrl+fileName);
//内容转换为对象
Type type = new TypeToken<List<Step>>(){}.getType();
//步骤集合
List<Step> list = gson.fromJson(content, type);
if(list == null){
list = new ArrayList<Step>();
}
boolean isDel = false;
for (int i = 0; i < list.size(); i++) {
if(list.get(i).getId() == id){
list.remove(i);
isDel = true;
}
}
if(isDel){
//内容写入到文件
JsonFileUtils.writeText(fileUrl+fileName,gson.toJson(list),false);
info.setData(list);
info.setMsg("删除步骤成功");
info.setCode(200);
}else{
info.setMsg("删除步骤失败,未找到步骤");
info.setCode(-203);
}
return info;
}
@Override
public Info delStep() {
Info info = new Info();
//读取文件内容
String content = JsonFileUtils.readText(fileUrl+fileName);
//内容转换为对象
Type type = new TypeToken<List<Step>>(){}.getType();
//步骤集合
List<Step> list = gson.fromJson(content, type);
if(list == null){
list = new ArrayList<Step>();
}
if(list.size() > 0){
//内容写入到文件 写一个空覆盖掉
JsonFileUtils.writeText(fileUrl+fileName,"",false);
list = new ArrayList<Step>();
info.setData(list);
info.setMsg("删除步骤成功");
info.setCode(200);
}else{
info.setMsg("已经没有内容了");
info.setCode(-205);
}
return info;
}
@Override
public Info runStep(RunInfo ri) {
Info info = new Info();
int runType = ri.getRunType();
//读取脚本
String content = JsonFileUtils.readText(fileUrl+fileName);
//内容转换为对象
Type type = new TypeToken<List<Step>>(){}.getType();
//步骤集合
List<Step> list = gson.fromJson(content, type);
if(list == null){
//不允许空运行
info.setCode(-206);
info.setMsg("脚本为空,请添加脚本过后继续运行");
return info;
}
if(runType == 1){//运行一次
//循环脚本步骤
try {
if(StepRun.isStepRun){
info.setCode(200);
info.setMsg("脚本正在运行中,请勿重复运行");
return info;
}
sr = new StepRun();
sr.setRunType(1);
sr.setList(list);
sr.run();
info.setCode(200);
info.setMsg("脚本运行成功,请在控制台查看运行状态");
} catch (Exception e) {
e.printStackTrace();
info.setCode(-207);
info.setMsg("脚本运行失败。");
return info;
}
}else if(runType == 2){//重复运行
if(StepRun.isStepRun){
info.setCode(200);
info.setMsg("脚本正在运行中,请勿重复运行");
return info;
}
sr = new StepRun();
sr.setRunType(2);
sr.setList(list);
sr.run();
info.setCode(200);
info.setMsg("脚本运行成功,请在控制台查看运行状态");
return info;
}else if(runType == 3){//定时运行
}
return info;
}
@Override
public Info runStop() {
try {
StepRun.isStepRun = false;
//中断线程
sr.interrupt();
MyWebSocketHandler.sendMsg(new MegInfo(200,"任务已停止"));
return new Info(200,"停止任务成功",null);
} catch (Exception e) {
return new Info(-200,"停止异常",null);
}
}
}
脚本线程
package com.sjy.automation.step;
import com.sjy.automation.bean.MegInfo;
import com.sjy.automation.bean.Step;
import com.sjy.automation.util.FileUtils;
import com.sjy.automation.ws.MyWebSocketHandler;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.List;
/**
* 和python通信完成后续脚本
*/
public class StepRun extends Thread{
//线程控制
public static boolean isStepRun = false;
private final static String fileUrl = FileUtils.getTomcatPath().substring(1)+"/upload/";
private static final String FILE = fileUrl+"xstx.py";
private static final String PYTHON = fileUrl+"python/pythonw.exe";
//数据
private List<Step> list;
public void setList(List<Step> list) {
this.list = list;
}
//运行类型
private int runType;
public void setRunType(int runType) {
this.runType = runType;
}
private Process proc;
@Override
public void run() {
try {
//循环调用并拿到结果
if(runType == 1){//只执行一遍
isStepRun = true;
int i = 0;
while (i < list.size() && isStepRun) {
String imgUrl = list.get(i).getImgurl();
if(imgUrl == null)
imgUrl = "null";
String content =list.get(i).getContent();
if(content == null)
content = "null";
Integer stepType = list.get(i).getStepType();
if(stepType == 5){
int i1 = Integer.parseInt(list.get(i).getContent());
MyWebSocketHandler.sendMsg(new MegInfo(200,"正在执行第"+(i+1)+"步,休息"+i1+"秒"));
Thread.sleep(i1 * 1000);
i++;
continue;
}
BufferedReader in = runStep(i, stepType, img2FileImg(imgUrl), content);
String line = null;
while ((line = in.readLine()) != null) {
MyWebSocketHandler.sendMsg(new MegInfo(200,line));
}
MyWebSocketHandler.sendMsg(new MegInfo(200,"第"+(i+1)+"步执行成功"));
in.close();
proc.waitFor();
i++;
}
MyWebSocketHandler.sendMsg(new MegInfo(200,""));
MyWebSocketHandler.sendMsg(new MegInfo(200,"第脚本任务执行完毕"));
isStepRun = false;
}else if(runType == 2){
isStepRun = true;
int i = 0;
int k = 1;
while (isStepRun) {
String imgUrl = list.get(i).getImgurl();
if(imgUrl == null)
imgUrl = "null";
String content =list.get(i).getContent();
if(content == null)
content = "null";
Integer stepType = list.get(i).getStepType();
if(stepType == 5){
int i1 = Integer.parseInt(list.get(i).getContent());
MyWebSocketHandler.sendMsg(new MegInfo(200,"正在执行第"+(i+1)+"步,休息"+i1+"秒"));
Thread.sleep(i1 * 1000);
}
BufferedReader in = runStep(i, stepType, img2FileImg(imgUrl), content);
String line = null;
while ((line = in.readLine()) != null) {
MyWebSocketHandler.sendMsg(new MegInfo(200,line));
}
MyWebSocketHandler.sendMsg(new MegInfo(200,"第"+(i+1)+"步执行成功"));
in.close();
proc.waitFor();
i++;
if(i == list.size()){
i = 0;
k++;
MyWebSocketHandler.sendMsg(new MegInfo(200,""));
MyWebSocketHandler.sendMsg(new MegInfo(200,"第"+k+"轮循环开始"));
}
}
}
} catch (IOException e) {
e.printStackTrace();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public BufferedReader runStep(int i,int type,String imgUrl,String content) throws IOException, InterruptedException {
MyWebSocketHandler.sendMsg(new MegInfo(200,"正在执行第"+(i+1)+"步"));
String typeStr = String.valueOf(type);
String[] args1 = new String[] {PYTHON, FILE,typeStr,imgUrl,content};//python.exe处为你系统中python的安装位置;python.py为想要执行的python文件位置;test为想要传的参数
proc=Runtime.getRuntime().exec(args1);
//用输入输出流来截取结果
BufferedReader in = new BufferedReader(new InputStreamReader(proc.getInputStream()));
Thread.sleep(100);
return in;
}
public static String img2FileImg(String url){
return url.replace("/image/",fileUrl+"image/").trim();
}
}
WebSocket模块
package com.sjy.automation.ws;
import com.google.gson.Gson;
import com.sjy.automation.bean.MegInfo;
import org.springframework.web.socket.*;
import java.io.IOException;
import java.util.concurrent.ConcurrentHashMap;
public class MyWebSocketHandler implements WebSocketHandler {
private static Gson gson = new Gson();
//保存用户链接
private static ConcurrentHashMap<String, WebSocketSession> users = new ConcurrentHashMap<String, WebSocketSession>();
// 连接 就绪时
@Override
public void afterConnectionEstablished(WebSocketSession session)
throws Exception {
users.put(session.getId(), session);
}
// 处理信息
@Override
public void handleMessage(WebSocketSession session,
WebSocketMessage<?> message) throws Exception {
System.err.println(session + "---->" + message + ":"+ message.getPayload().toString());
}
// 处理传输时异常
@Override
public void handleTransportError(WebSocketSession session,
Throwable exception) throws Exception {
}
// 关闭 连接时
@Override
public void afterConnectionClosed(WebSocketSession session,
CloseStatus closeStatus) {
System.out.println("用户关闭页面");
users.remove(session.getId());
}
//是否支持分包
@Override
public boolean supportsPartialMessages() {
return false;
}
public static void sendMsg(MegInfo mi) throws IOException {
for (String s : users.keySet()) {
WebSocketSession wss = users.get(s);
wss.sendMessage(new TextMessage(gson.toJson(mi)));
}
}
}
Info类
package com.sjy.automation.bean;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.stereotype.Component;
@Data
@AllArgsConstructor
@NoArgsConstructor
@Component
public class Info {
private Integer code;//状态码
private String msg;//信息
private Object data;//数据
}
最后修改于 2022-01-07 10:42:25
如果觉得我的文章对你有用,请随意赞赏
扫一扫支付
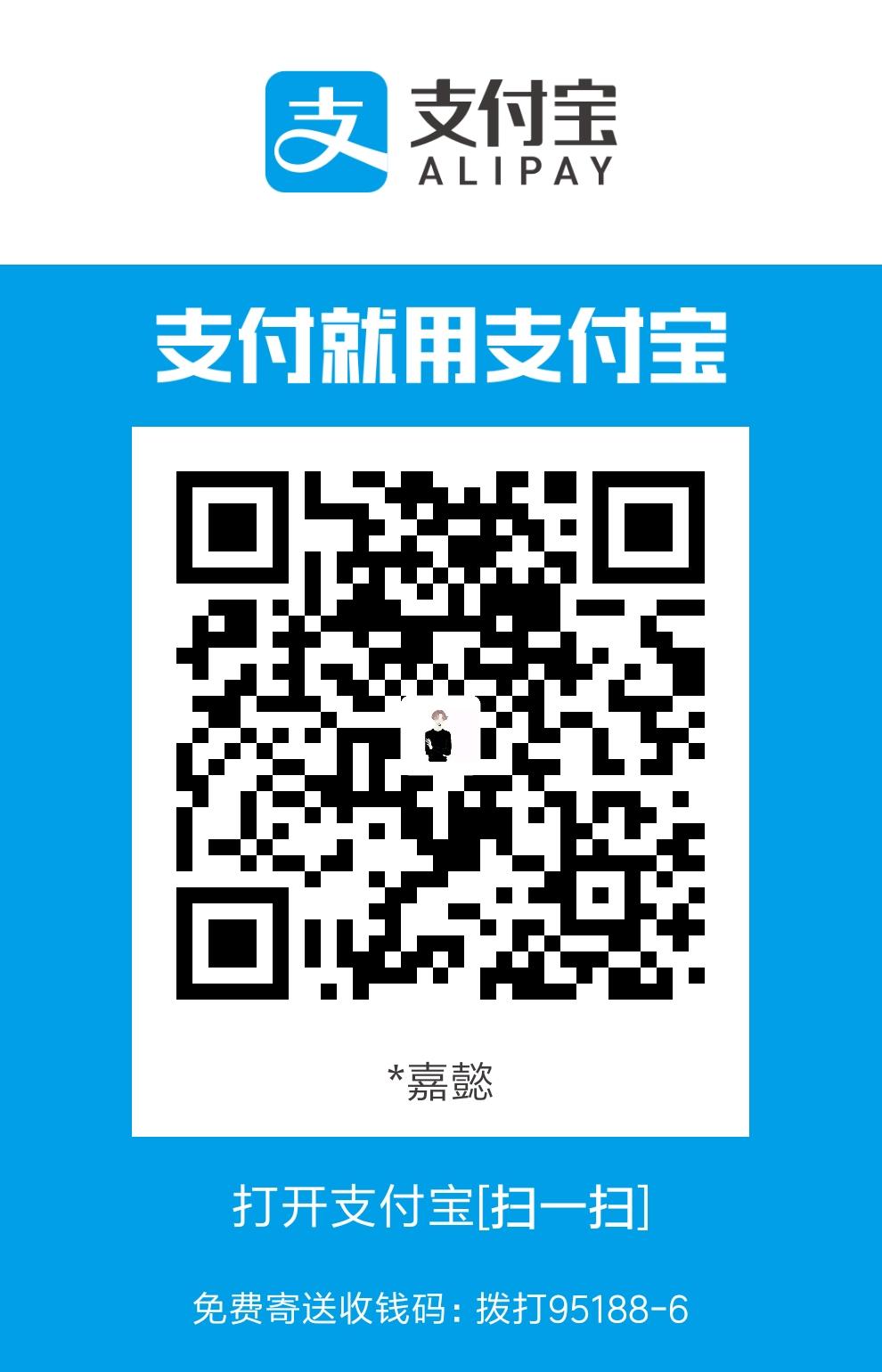
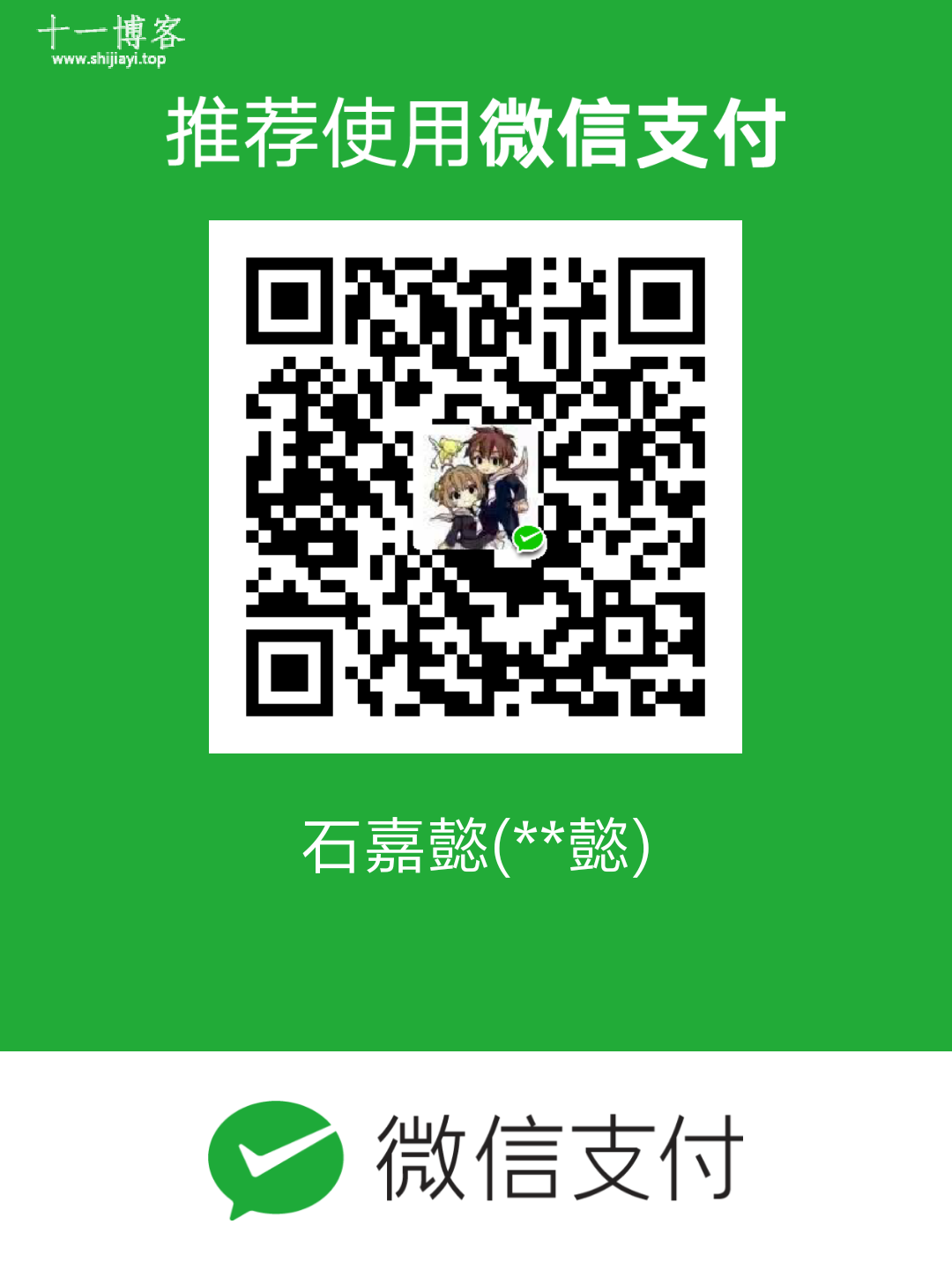