index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<title>hellovue</title>
<link rel="stylesheet" type="text/css" href="static/layui/css/layui.css"/>
<script src="static/layui/layui.js" type="text/javascript" charset="utf-8"></script>
<!-- 弹幕插件 -->
<link rel="stylesheet" type="text/css" href="static/css/barrager.css"/>
<script src="http://libs.baidu.com/jquery/2.0.3/jquery.min.js"></script>
<script src="static/js/jquery.barrager.min.js" type="text/javascript" charset="utf-8"></script>
<style>
body{
background-color: black;
}
</style>
</head>
<body>
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
Message.vue
<template>
<div>
<div class="msg-title">
相亲相爱一家人({{uNumber}}人在线)
</div>
<div class="msg-content" ref="message">
<p v-if="initMsg">{{initMsg}}</p>
<ul>
<li v-for="(msg,index) in msgList" :key="index">
<template v-if="msg.code == 1">
<span>{{msg.sName}}</span><span>{{msg.time}}</span>
<p style="color:green;">{{msg.msg}}</p>
</template>
<template v-if="msg.code == 4">
<span>{{msg.sName}}</span><span>{{msg.time}}</span>
<p style="color:red;">{{msg.msg}}</p>
</template>
<!-- template 不会显示在页面上 一般搭配if使用 -->
<template v-if="msg.code == 2">
<span>{{msg.time}}</span>
<p>{{msg.sName}}<b>说:</b>
<!-- <span v-html="msg.msg"></span> -->
<span>{{msg.msg}}</span>
</p>
</template>
<br>
</li>
</ul>
</div>
<div class="send-msg">
<textarea placeholder="请输入信息" class="layui-textarea send-con" v-model="message" @keydown.enter="sendMsg"></textarea>
<button type="button" class="layui-btn send-btn" @click="sendMsg">
<i class="layui-icon"></i>
发送(Enter)
</button>
</div>
</div>
</template>
<script>
import pubsub from 'pubsub-js';
export default {
name:'Message',
data (){
return {
message:"",
initMsg:"",
msgList:[],
uNumber:0
}
},
methods:{
sendMsg(){
if(this.message === ""){
alert("发送信息不能为空");
}else{
//发给APP组件,让APP组件发给服务器
pubsub.publish('sendMsg',this.message);
this.message = "";
}
}
},
mounted(){//所有元素加载完成之后调用
//创建一个消息接收者
this.pub = pubsub.subscribe('init',(nsgName,data) => {
this.initMsg = data;
});
//接收信息
this.newUser = pubsub.subscribe('newUser',(nsgName,data) => {
// {"code":1,"msg":"周攀连接上来了","sName":"系统","time":"2021-10-13 14:57:49"}
//在数组后面追加对象
this.msgList.push(data);
setTimeout(() => {
//滚动条在最底部 设置滚动条的当前高度为最大高度
this.$refs.message.scrollTop = this.$refs.message.scrollHeight;
}, 500);
});
//接受总人数
this.userNumber = pubsub.subscribe('userNumber',(nsgName,data) => {
this.uNumber = data;
});
},
beforeDestroy(){//组件摧毁时触发
//摧毁掉消息接收者,提高性能
pubsub.unsubscribe(this.pub);
pubsub.unsubscribe(this.newUser);
pubsub.unsubscribe(this.userNumber);
}
}
</script>
<style>
/* 控制图片大小 */
img{
width: 200px;
height: 200px;
}
.msg-title{
width: 700px;
height: 50px;
float: left;
text-align: center;
line-height: 50px;
font-size: 25px;
background-color: #ffffff;
}
.msg-content{
width: 700px;
height: 650px;
/* 垂直滚动条 */
overflow-y: auto;
float: left;
/* 强制换行 */
word-wrap: break-word;
background-color: #FFD7BA;
}
/*滚动条样式*/
.msg-content::-webkit-scrollbar {/*滚动条整体样式*/
width: 4px; /*高宽分别对应横竖滚动条的尺寸*/
height: 4px;
}
.msg-content::-webkit-scrollbar-thumb {/*滚动条里面小方块*/
border-radius: 5px;
-webkit-box-shadow: inset 0 0 5px rgba(0,0,0,0.2);
background: rgba(0,0,0,0.2);
}
.msg-content::-webkit-scrollbar-track {/*滚动条里面轨道*/
-webkit-box-shadow: inset 0 0 5px rgba(0,0,0,0.2);
border-radius: 0;
background: rgba(0,0,0,0.1);
}
.send-msg{
width: 700px;
height: 200px;
float: left;
background-color: #FEC89A;
}
.send-btn{
position: relative;
left: 557px;
bottom: 50px;
}
.send-con{
height: 200px;
position: relative;
}
</style>
UserList.vue
<template>
<div class="user-list">
<ul class="layui-menu" style="margin-top: 0px;">
<li v-for="ul in userList" :key="ul.userId">
<div class="layui-menu-body-title">{{ul.userName}}</div>
</li>
</ul>
</div>
</template>
<script>
import pubsub from 'pubsub-js';
export default {
name:'UserList',
data(){
return{
userList:[]
}
},
mounted(){
//收到用户列表
this.userList = pubsub.subscribe('userList',(nsgName,data) => {
this.userList = data;
});
},
beforeDestroy(){//组件摧毁时触发
//摧毁掉消息接收者,提高性能
pubsub.unsubscribe(this.userList);
}
}
</script>
<style>
.user-list{
width: 300px;
height: 900px;
float: left;
overflow-y: auto;
overflow-x: hidden;
background-color: #FAE1DD;
}
/*滚动条样式*/
.user-list::-webkit-scrollbar {/*滚动条整体样式*/
width: 4px; /*高宽分别对应横竖滚动条的尺寸*/
height: 4px;
}
.user-list::-webkit-scrollbar-thumb {/*滚动条里面小方块*/
border-radius: 5px;
-webkit-box-shadow: inset 0 0 5px rgba(0,0,0,0.2);
background: rgba(0,0,0,0.2);
}
.user-list::-webkit-scrollbar-track {/*滚动条里面轨道*/
-webkit-box-shadow: inset 0 0 5px rgba(0,0,0,0.2);
border-radius: 0;
background: rgba(0,0,0,0.1);
}
</style>
App.vue
<template>
<div id="app" class="box">
<UserList/>
<Message/>
</div>
</template>
<script>
//引入组件
import UserList from './components/UserList.vue';
import Message from './components/Message.vue';
import pubsub from 'pubsub-js';
export default {
name: 'App',
components: {//注册组件
UserList,
Message
},
mounted(){//当所有DOM元素渲染完成之后会调用
// ws:// ip地址 / 接口名称
var ws = new WebSocket("ws://192.168.1.188/ChatServer/张三");
ws.onopen = function(){//通道建立时触发
//通过pubsub 消息订阅插件发给mseeage组件 npm install pubsub-js -S
pubsub.publish('init',"与聊天服务器连接成功");
}
ws.onmessage = function(event){//收到服务器发送的信息时触发
//json字符串转对象
var data = JSON.parse(event.data);
if(data.code == 1){//有新人连上来的信息
pubsub.publish('newUser',data);//把收到的信息发送给message组件
}else if(data.code == 2){//收到聊天信息
pubsub.publish('newUser',data);
//js去除标签
var temp = data.msg.replace(/<\/?.+?>/g, "");
var result = temp.replace(/ /g, "");//result为得到后的内容
//生成一个随机数,用来控制弹幕的速度
var speedRan = Math.ceil(Math.random()*10);
var item={
img:'static/imgs/1.jpeg', //图片
info:result, //文字
href:'#', //链接
close:true, //显示关闭按钮
speed:speedRan, //延迟,单位秒,默认6
// bottom:70, //距离底部高度,单位px,默认随机
color:'#fff', //颜色,默认白色
old_ie_color:'#000000', //ie低版兼容色,不能与网页背景相同,默认黑色
}
//控制当弹幕量过大的处理
if($(".barrage").length < 30){
$('body').barrager(item);
}
}else if(data.code == 3){//聊天室总人数
pubsub.publish('userNumber',data.uNumber);
}else if(data.code == 4){//下线通知
pubsub.publish('newUser',data);
}else if(data.code == 5){//收到了用户列表
console.log(data.userMap);
pubsub.publish('userList',data.userMap);
}
}
ws.onclose = function(){//连接断开时触发
}
ws.onerror = function(){//通道异常时触发
}
//接收消息组件发送给App组件
this.sendMsg = pubsub.subscribe('sendMsg',(nsgName,data) => {
//页面端websocket发送消息
ws.send(data);
});
//当页面关闭时主动断开连接
window.onbeforeunload = function(){
ws.close();
}
},
beforeDestroy(){//组件摧毁时触发
//摧毁掉消息接收者,提高性能
pubsub.unsubscribe(this.sendMsg);
}
}
</script>
<style scoped>
body{
background-color: #000000;
}
.box{
width: 1000px;
height: 900px;
margin: 0 auto;
background-color: #5FB878;
}
</style>
最后修改于 2021-10-14 15:30:22
如果觉得我的文章对你有用,请随意赞赏
扫一扫支付
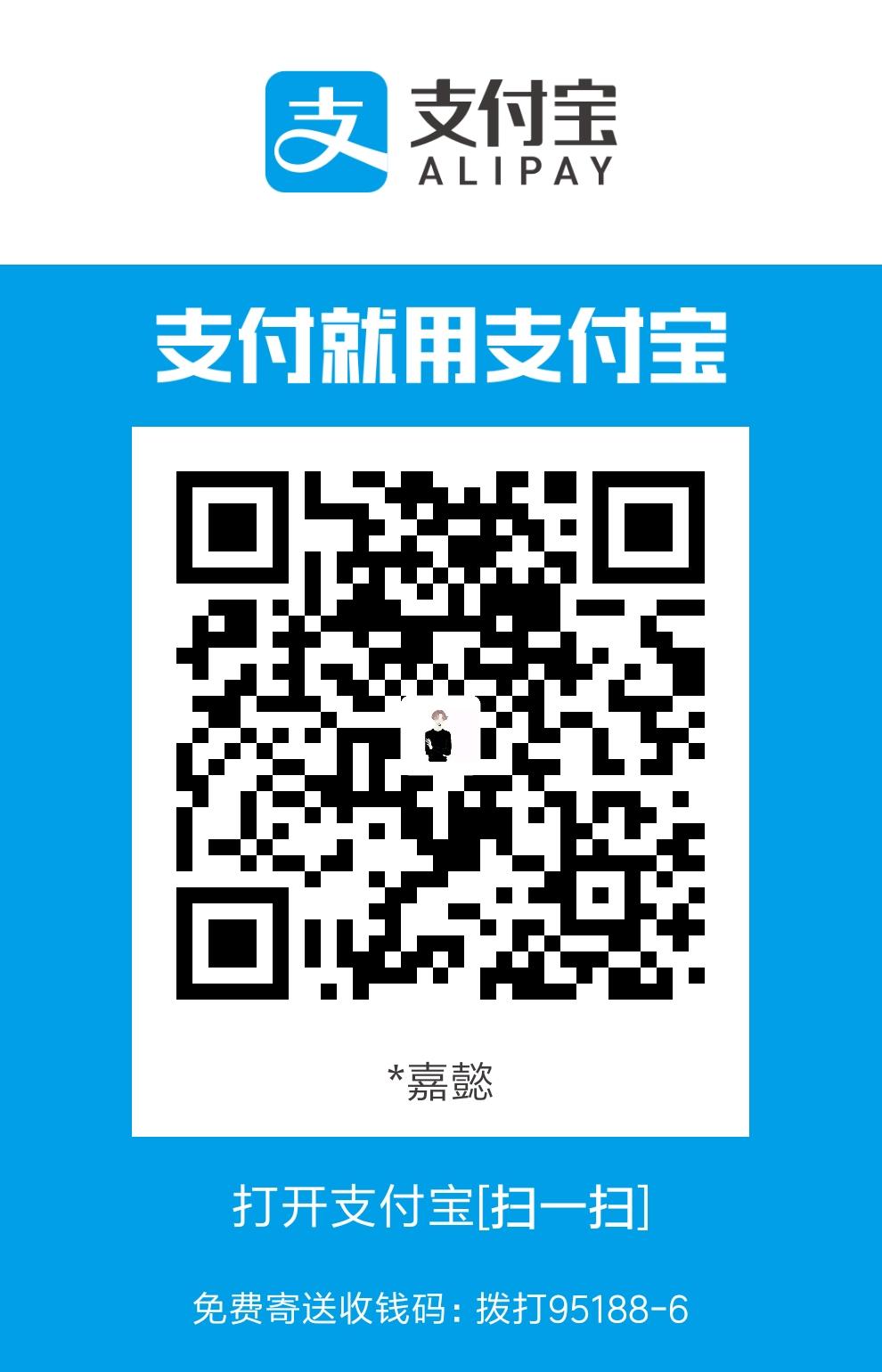
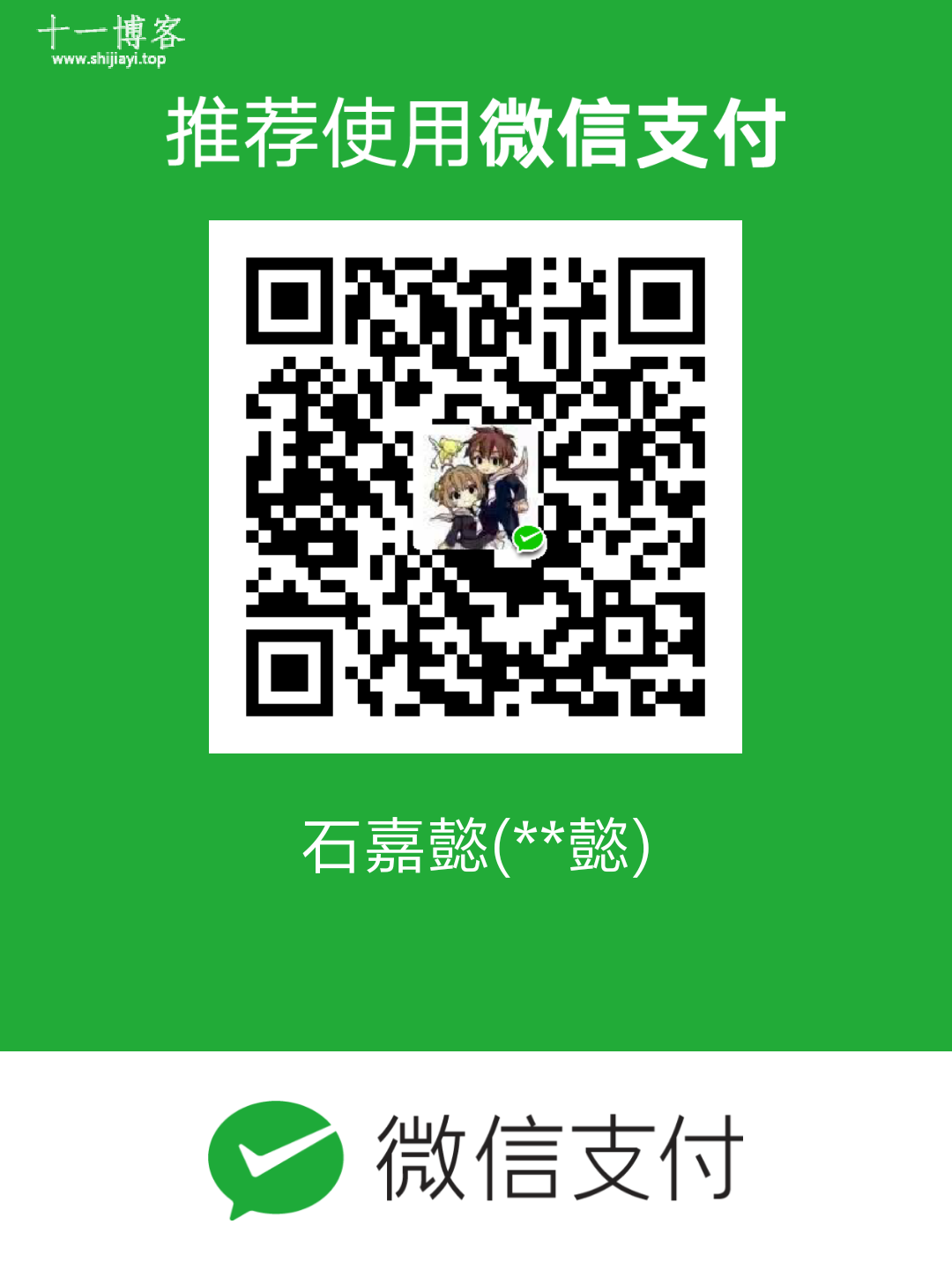