1、Mat转byte
private static int[] mat2RGB(Mat src) {
int width = src.cols();
int height = src.rows();
int dims = src.channels();
byte[] data = new byte[width * height * dims];
src.get(0, 0, data);
int[] pixels = new int[width * height];
BufferedImage bi = new BufferedImage(width,height,BufferedImage.TYPE_INT_ARGB);
int r = 0, g = 0, b = 0;
int index = 0;
for(int row = 0; row < height; row++) {
for(int col = 0; col < width; col++) {
index = row * width * dims + col * dims;
if(dims == 3) {
b = data[index]&0xff;
g = data[index+1]&0xff;
r = data[index+2]&0xff;
pixels[row * width + col] = ((255&0xff) << 24) | ((r&0xff) << 16) | ((g&0xff) << 8) | (b&0xff);
}else if(dims == 1) {
b = data[index]&0xff;
pixels[row * width + col] = ((255&0xff) << 24) | ((b&0xff) << 16) | ((b&0xff) << 8) | (b&0xff);
}
}
}
return pixels;
}
2、int[]转byte[]
private static byte[] rgbToGray(int[] argb, int width, int height) {
int yIndex = 0;
int index = 0;
byte[] gray = new byte[width * height];
for(int j = 0; j < height; ++j) {
for(int i = 0; i < width; ++i) {
int R = (argb[index] & 16711680) >> 16;
int G = (argb[index] & '\uff00') >> 8;
int B = argb[index] & 255;
int Y = (66 * R + 129 * G + 25 * B + 128 >> 8) + 16;
gray[yIndex++] = (byte)(Y < 0 ? 0 : (Y > 255 ? 255 : Y));
++index;
}
}
return gray;
}
经过测试,每帧运行速度比原来提高了0.75
最后修改于 2021-01-28 14:54:26
如果觉得我的文章对你有用,请随意赞赏
扫一扫支付
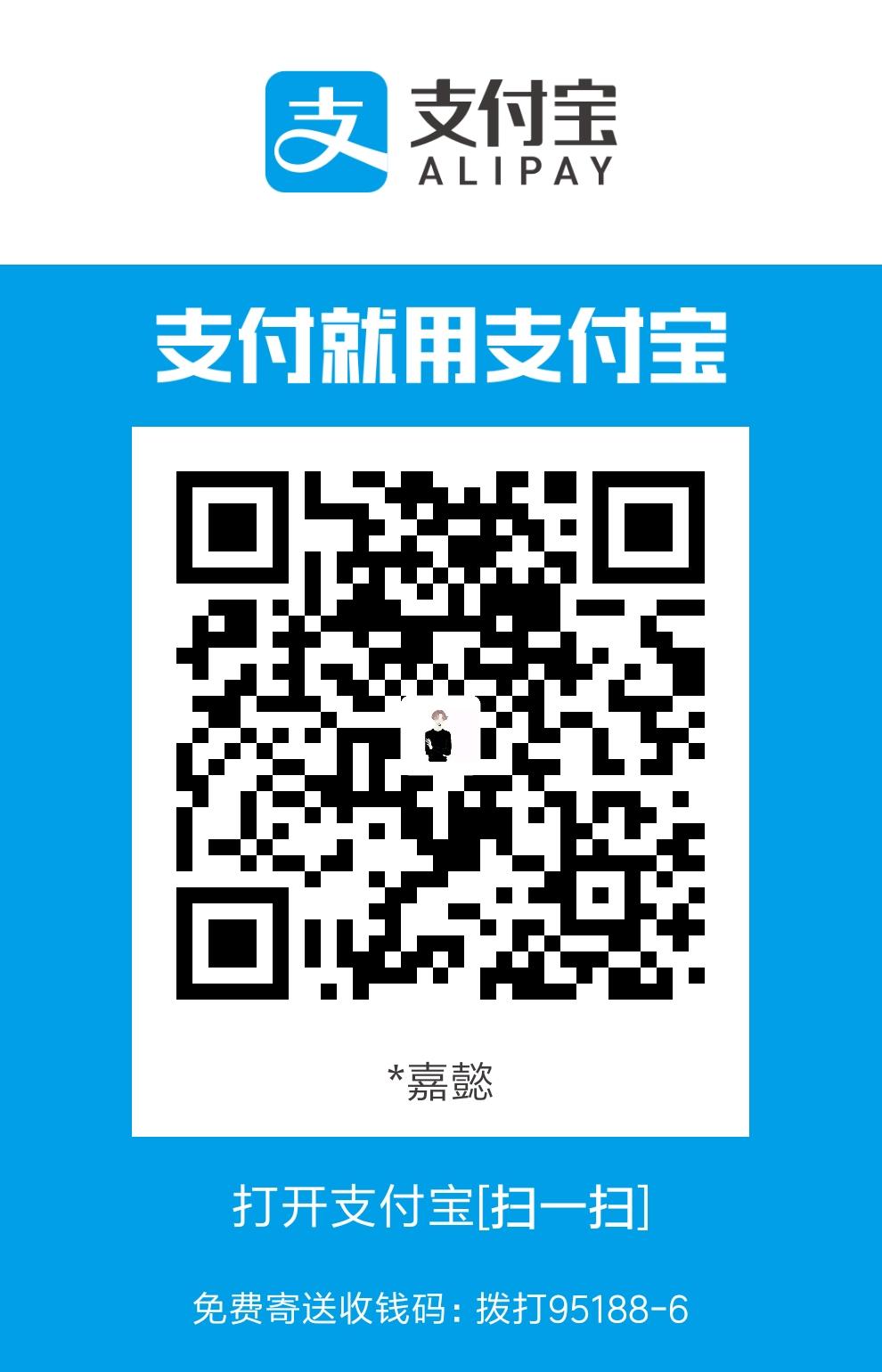
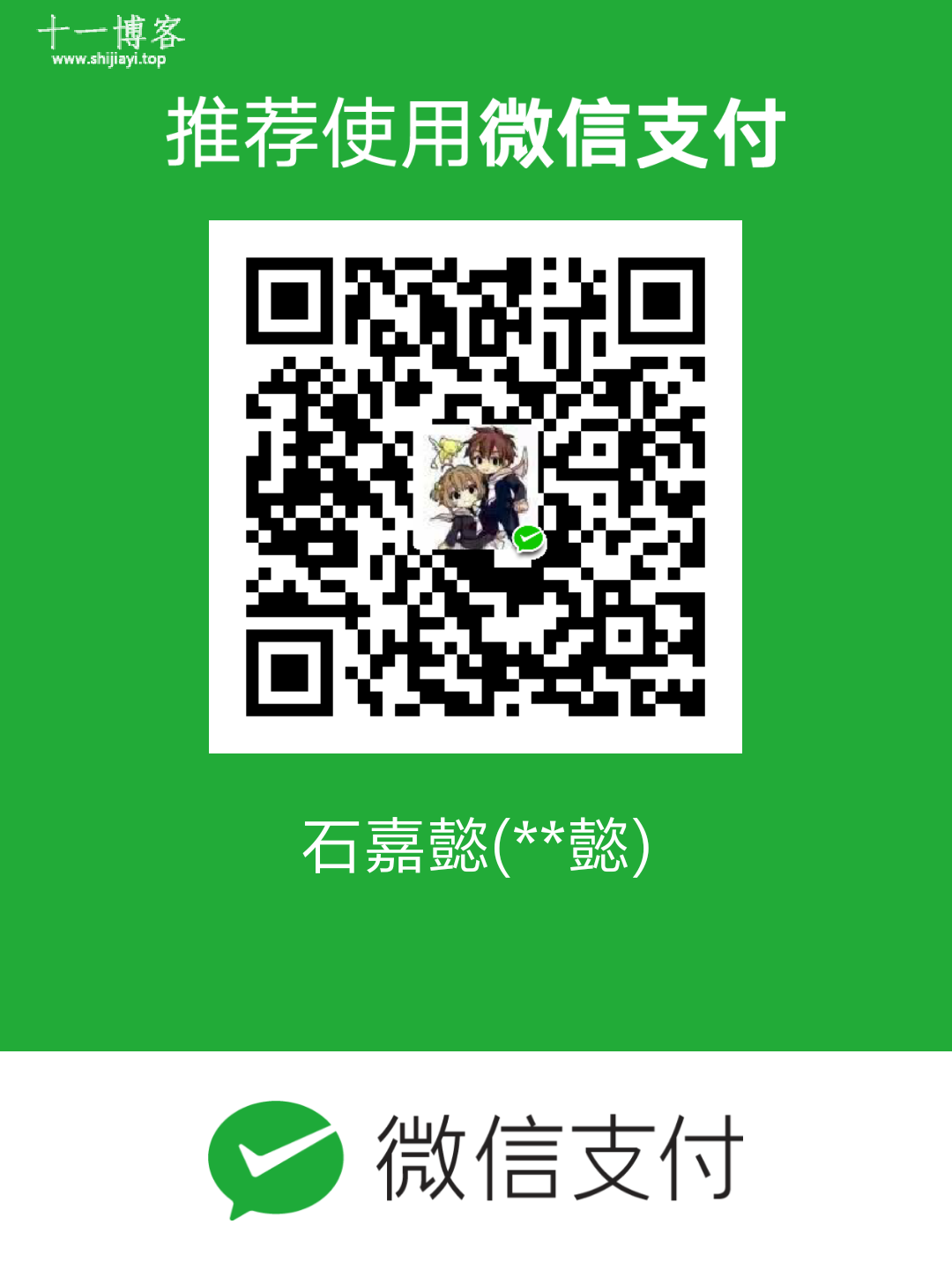